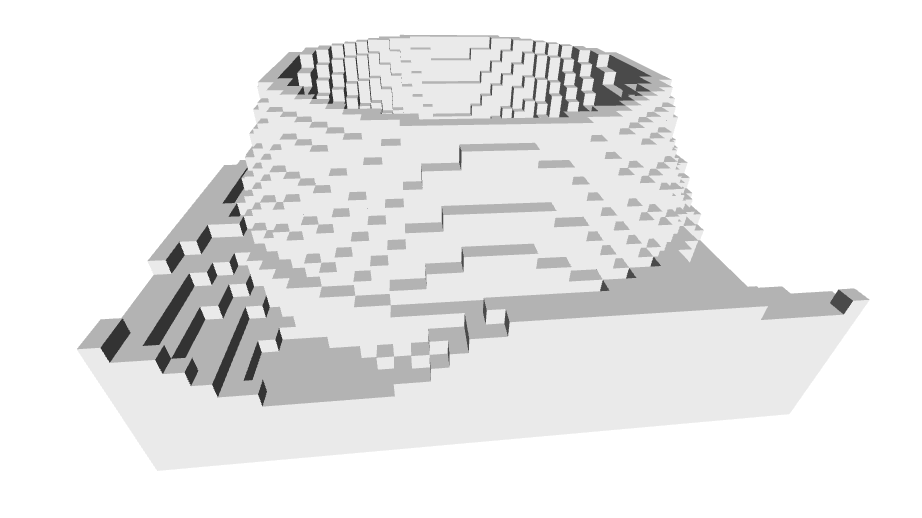
Creating 3D-printable landscapes and craters in Logo
Terrain can be saved as STL files (under the File menu in the web IDE) and then 3D printed!
setterrain
[x1 y1 x2 y2] (list) | [height floor ceiling] (list) | seed (number) | algorithm (word) OR [algorithm magnification] | style (word)
Creates terrain on the z-plane between the specified x and y co-ordinates.
These co-ordinates represent 10 turtle-units, and the output can be scaled using setterrainresolution.
height specifies the maximum height in terms of single turtle-units, similarly scaled using setterrainresolution. The floor value specifies the lowest level rendered, and any values lower than that value are rendered at the floor level. Similarly, the ceiling value represents the highest value rendered, and anything higher is lowered to that value.
The seed is provided to the algorithm that generates the terrain, the algorithm is the method used (currently only fbm and flat (no algorithm) are supported) and the style (voxel, line, triangle or combo (line and triangle) — note that combo uses the terraininterior color to render its lines).
Providing a list containing the algorithm type and a multiplier allow you to ‘zoom in’ or out on the terrain, making it more dense and complex, or spacious and simple. Multiple blocks of terrain can be defined so long as they do not overlap.
See terrain, setterrainresolution, setterraincolors, setterraininterior, setelevation, elevation, lockelevation, elevationsnap, unlockelevation
setterrain [-10 -10 10 10] [25] 25 “fbm “voxel
setterrainresolution
number
Sets the ‘resolution’ of the terrain, or the size of each ‘sector’ in turtle-units within the terrain ‘grid’ which expands out from [0 0]. For example, the higher the terrainresolution is the larger each voxel is in the terrain in the voxel mode, and the more area the terrain covers. See setterrain, terrainresolution
setterrainreolution 5
setelevation
[x y elevation] list OR [[x y elevation][x2 y2 elevation2]…]]
Sets the elevation height of the terrain at the specified x and y co-ordinates. If a floor value is set in the terrain impacted by setelevation, the elevation will be set to the floor value if it is greater than the elevation specified, unless the new elevation is provided as a list of a single value.
setelevation can also take list of lists. See elevation, setterrain
setelevation [10 20 10]
Voxel-based terrain generation:
TO voxelterrain reset hideturtle setterrainresolution 5 setterrain [-2 -2 2 2] [20 1 10] random 500 [fbm 2] "voxel END
Triangle-based terrain generation:
TO triangleterrain reset hideturtle setterrainresolution 5 setterrain [-2 -2 2 2] [20 1 10] random 500 [fbm 2] "triangle ;the only real difference is changing the last parameter above to 'triangle' END
Voxel-based crater generation:
TO voxelcrater reset hideturtle setterrainresolution 5 setterrain [-2 -2 2 2] [20 1 10] random 500 [fbm 2] "voxel stop dropanchor penup pullout 50 repeat 10 [ repeat 180 [ orbitright 2 setelevation {xpos ypos 40 + 5 * repabove 1} ] pullout 2 ] pullout 2 repeat 9 [ repeat 180 [ orbitright 2 setelevation {xpos ypos 95 - 5 * repabove 1} ] pullout 2 ] END
Triangle-based crater generation:
TO trianglecrater reset hideturtle setterrainresolution 5 setterrain [-2 -2 2 2] [20 1 10] random 500 [fbm 2] "triangle stop dropanchor penup pullout 50 repeat 10 [ repeat 180 [ orbitright 2 setelevation {xpos ypos 40 + 5 * repabove 1} ] pullout 2 ] pullout 2 repeat 9 [ repeat 180 [ orbitright 2 setelevation {xpos ypos 95 - 5 * repabove 1} ] pullout 2 ] END