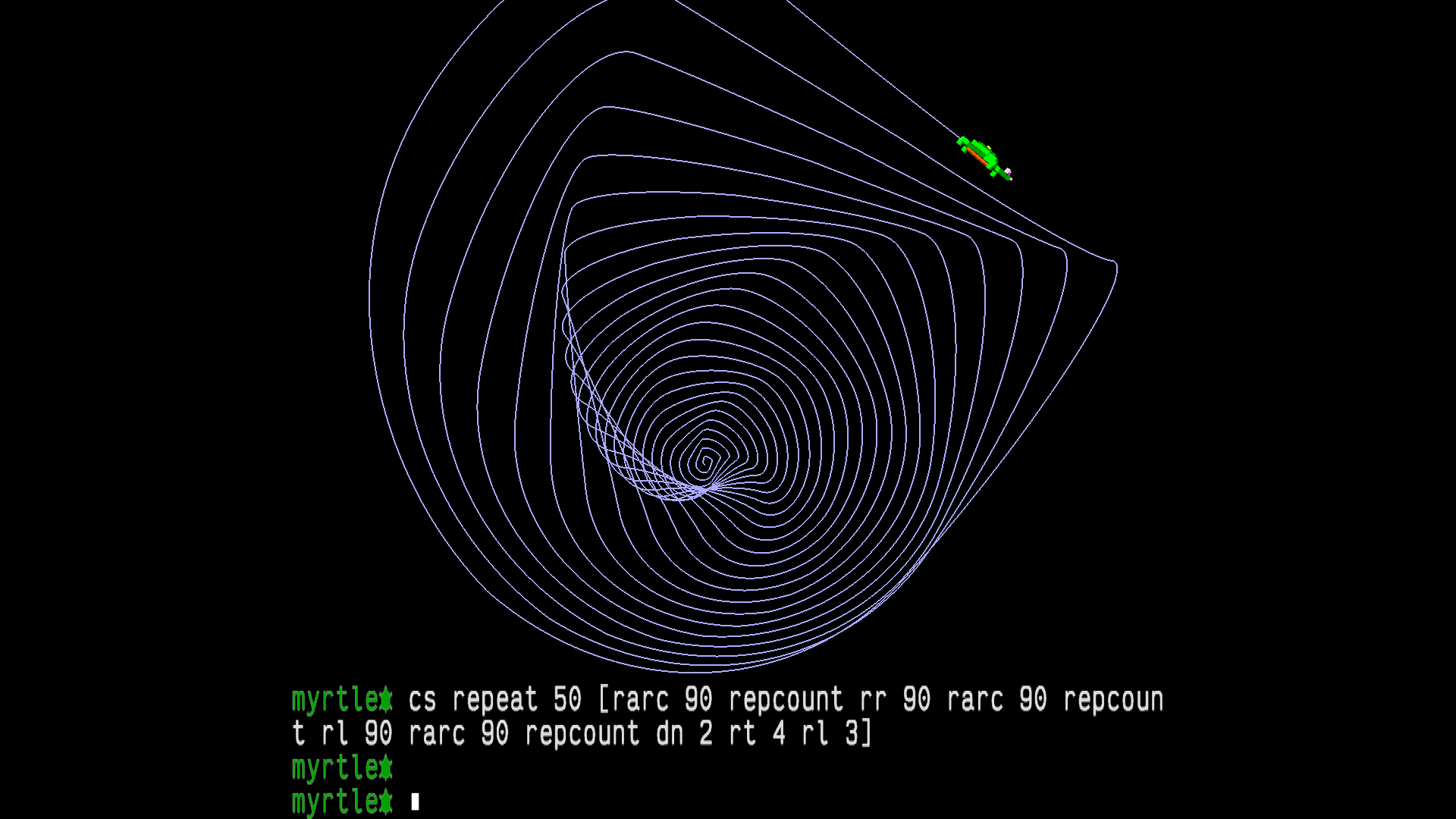
Quick Play: Warped Logo Spirals
One of the best things about turtleSpaces is its capacity for discovery through play.
Today I was playing with spirals:
I started off by creating a simple spiral using the RARC primitive and a simple REPEAT loop. This loop uses REPCOUNT (the current repeat loop iteration) to define the radius of the arc, resulting in an ever-growing spiral.
I clicked and dragged on the window to rotate the camera around the spiral. But let’s make the spiral itself three-dimensional:
I’ve ‘warped’ the spiral by placing three rarcs inside of the repeat loop, along with a ROLLLEFT (rl) and a ROLLRIGHT (rr). With the twisting and turning, the turtle ends up roughly halfway around a somewhat distorted circle, which you can see if you type:
cs rarc 90 20 rr 90 rarc 90 20 rl 90 rarc 90 20 cs repeat 2 [rarc 90 20 rr 90 rarc 90 20 rl 90 rarc 90 20]
If we orbit the camera (by clicking and dragging) around our spiral, we can see just how warped it is!
What else can we do with it?
Whoa! What happened there? I added a dn (down) 10 to the end of the loop. Let’s take a look from another vantage point:
That’s pretty distorted. Let’s try a smaller value, say 2:
And what about 5?
What about down (dn) instead of up?
Okay, pretty neat. How about if at the end of each loop we also roll left (rl) a little?
Another angle:
Let’s play around with the values of down (dn) and rollleft (rl) a bit:
What if we do a right turn (rt) instead of a roll right (rr)?
And what if we add the roll back in?
Let’s play around with the values a bit:
All right, that’s enough from me! But you can play around with the different values and see what happens. That’s all part of the fun of turtleSpaces!