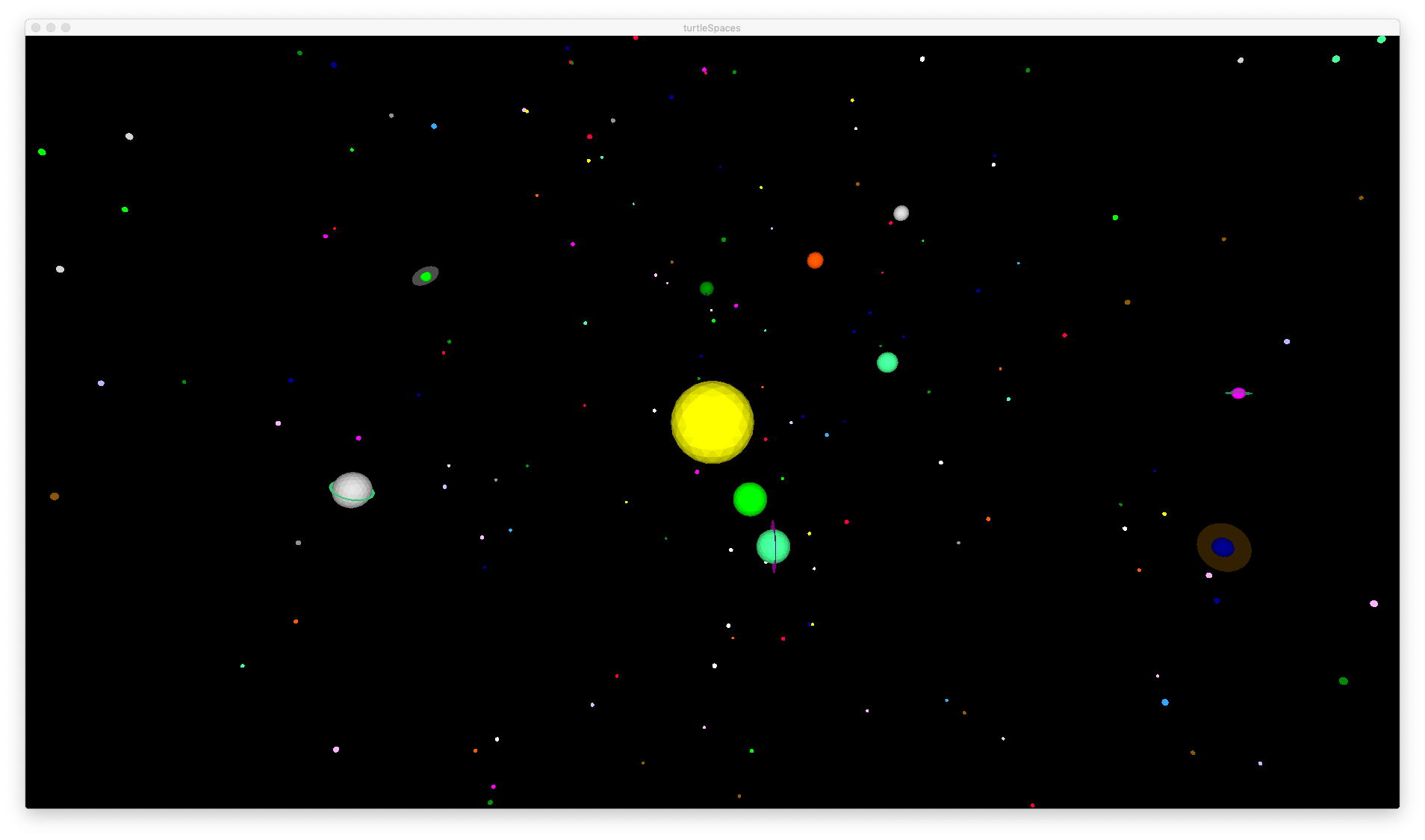
Example: Random Solar System
Create a ‘random’ solar system and surrounding star field using these commented Logo procedures:
TO solsys reset ;reset the turtles and clear the environment fullscreen ;don't display text ask "snappy [pullout 1000] ;ask the camera turtle to pull away from ;myrtle 1000 turtle units penup ;raise the turtle's pen so she doesn't draw hideturtle ;go incognito stars ;jump to the stars procedure, which creates ;a starfield around our solar system setfillcolor yellow icosphere 75 ;make the sun, an icosphere 75 turtle units ;in radius dropanchor ;set Myrtle's 'anchor point' or the point ;she orbits around to her home position. ;By default it is somewhat in front of it. pullout 100 ;pull out from the anchor point 100 turtle units repeat 10 [ ;do the following ten times, to create ten planets pullout 100 ;pull out from the anchor point 100 more ;turtle units orbitleft random 360 ;orbit to the left between 0 and 360 degrees ;(up to one full orbit of the anchor point) planet repcount ;jump to the planet procedure, passing it ;the current iteration of the repeat loop ;as its parameter - the current planet number ] ;this is the end of the planet creation loop snappy:forever [orbitdown 0.001] ;orbit around the scene ;snappy: is shorthand for 'ask "snappy []' END TO stars ;create a starfield repeat 500 [ randomvectors ;points the turtle in a random 3D ;direction. 'Vectors' describe absolute ;directions in 3D space using numbers. ;We call them vectors so as to not confuse ;them with relative directions such as up, ;down, left or right. A turtle with certain ;vectors will always point a certain way. ;For now, that's all you need to know! forward 1500 + random 500 ;move forward 1500 turtle units plus ;between 0 and 499 turtle units in the ;turtle's forward direction - its forward ;'vector' ;Note: 'random' generates a number between ;0 and one less than the number you give it. ;The turtle knows what you mean when you say ;'random' because random is a primitive - a ;word the turtle knows. up 90 ;tilt the turtle up 90 degrees, one quarter of ;a circle or one quarter of a complete rotation. ;Like as if you leaned back so far you were ;staring up at the sky randomfillcolor ;random means 'pick one at random', in this ;case a random fill color, numbered between 1 ;and 15 (there are 16 default colors, but 0 is ;transparent and not typically a useful fill ;color!) spot 5 + random 5 ;make a 'star', a spot with a radius of 5 ;turtle units plus 0 - 4 additional turtle units home ;go back to the home position ] ;make 500 stars END TO planet :number ;make a planet. The number passed to ;planet is used to uniquely identify ;its 'model' - what it looks like newmodel :number [ randomfillcolor ;Remember: not black! icosphere 10 + random 25 ;create a randomly-sized sphere randomvectors ;'what's your vectors, Victor?' dountil fillcolor != yellow [randomfillcolor] ;not yellow! spot 10 + random 30 ;create a randomly-sizes spot. ;If it's bigger than the sphere ;then it will act as a ring ] ;make a random planet model with the ;name of the planet number stored ;in the :number container, which was ;created when the number was passed ;in to the planet procedure ;Models are not unique to turtles, and ;so they each need a unique name, regardless ;of whatever turtle 'wears' them. But that ;name can just be a number, as long as it ;is a unique number hatch [ ;hatch a new turtle from this spot showturtle ;show the hatchling's model. Hatchlings ;models are hidden when they are hatched ;as are those of other turtles when they ;are newly created. Only Myrtle is shown ;by default put 1 + random 10 in "speed ;generate a random number between ;0 and 9, add it to 1 and put it in ;a container named 'speed' setmodel :number ;set the hatchling's 'model' or ;appearance to the name stored in ;the :number container setanchor [0 0 0] ;set the 'anchor' or the point a turtle ;orbits around to [0 0 0], the location ;of the sun forever [ orbitleft :speed * 0.001 ] ;orbit around the sun 'forever' ] ;this is the end of the hatchling's code END